集合介绍与API
声明一个set
let s = new Set();
let s2 = new Set(['1','2','3','4'])
console.log(s2)
元素个数
console.log(s2.size)
添加新的元素
s2.add('5')
console.log(s2)
删除元素
s2.delete('4')
检测
s2.has('1')
清空
s2.clear()
遍历
for(let v of s2){
console.log(v)
}
集合实践
let arr = [1,2,3,4,5,4,3,2,1]
let result = [...new Set(arr)]
console.log(result)
let arr2 = [4,5,6,5,6,]
let result = [...new Set(arr)].filter(item => {
let s2 = new Set(arr2)
if(s2.has(item)){
return true
}else{
return false
}
})
console.log(result)
let result = [...new Set([...arr, ...arr2])]
let diff = [...new Set(arr)].filter(item => !(new Set(arr2).has(item)))
Map
声明一个Map
let m = new Map()
添加元素
m.set('name','尚硅谷')
let key = {
school:'heiheihei'
}
m.set(key,['bj','sh','shenzhen'])
console.log(m)
console.log(m.size)
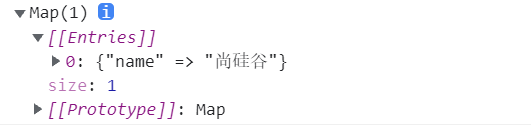
删除
m.delete('name')
获取
console.log(m.get('name'))
清空
m.clear()
遍历
for(let v of m){
console.log(v)
}
Class
ES5
function Phone(brand,price){
this.brand = brand
this.price = price
}
Phone.prototype.call = function(){
console.log('我可以打电话')
}
let Huawei = new Phone('华为',5999)
Huawei.call()
console.log(Huawei)
ES6
class Phone{
constructor(brand,price){
this.brand = brand
this.price = price
}
call(){
console.log('我可以打电话')
}
}
let oneplus = new Phone('1+',1999)
console.log(onePlus)
ES5构造函数继承
function Phone(brand,price){
this.brand = brand
this.price = price
}
Phone.prototype.call = function(){
console.log('我可以打电话')
}
function SmartPhone(brand,price,color,size){
Phone.call(this,brand,price)
this.color = color
this.size = size
}
SmartPhone.prototype == new Phone
SmartPhone.prototype.constructor = SmartPhone
SmartPhone.prototype.photo = function(){
console.log('我可以拍照')
}
Smartphone.prototype.playGame = function(){
console.log('我可以玩游戏')
}
const chuizi = new SmartPhone('锤子','2499','黑色','5.5inch')
console.log(chuizi)
class类继承
class Phone{
constructor(brand,price){
this.brand = brand
this.price = price
}
call(){
console.log('我可以打电话')
}
}
class SmartPhone extends Phone{
constructor(brand,price,color,size){
super(brand,price)
this.color = color
this.size = size
}
photo(){
console.log('拍照')
}
playGame(){
console.log('玩游戏')
}
}
const xiaomi = new SmartPhone('小米',799,'黑色','4.')